Ptychography Reconstruction¶
This notebook demonstrates a simulated ptychographic reconstruction using tike.
[1]:
import logging
import matplotlib.pyplot as plt
import numpy as np
import tike
import tike.ptycho
import tike.view
[2]:
for module in [tike, np]:
print("{} is version {}".format(module.__name__, module.__version__))
tike is version 0.15.2.dev39+g7ca618d.d20210126
numpy is version 1.19.5
Load test data¶
This data was simulated using tike and it used for continuous integration testing.
[3]:
import lzma
import pickle
with lzma.open('../../../tests/data/ptycho_setup.pickle.lzma', 'rb') as file:
[
data,
scan,
probe,
original,
] = pickle.load(file)
Define the object¶
The object consists of two unique views of varying phase: one with constant amplitude and one with varying amplitude.
[4]:
original.shape
[4]:
(2, 128, 128)
[5]:
# Center the phase image in the range (-pi, pi)
original = original * np.exp(-1j * np.pi/2)
[6]:
for i in range(len(original)):
plt.figure()
tike.view.plot_phase(original[i], amax=1, amin=0)
plt.show()
(0.001338858+0.9999991j) (1+0.00020559915j)
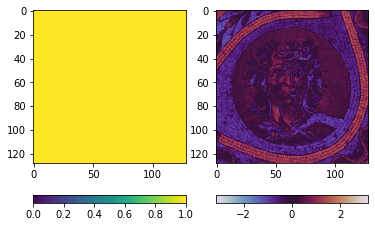
(0.00013624692+5.2331194e-05j) (0.9566685+0.0636505j)
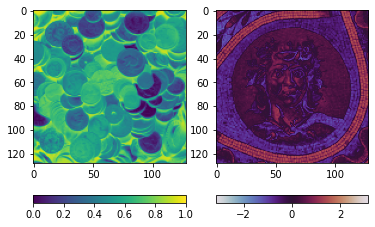
Define the probe¶
Note that the shape of the probe includes many dimensions. These dimensions are for providing multiple incoherent probes and coherent probbes along with unique probes for multiple dimensions. Read the documentation of the tike.ptycho.probe
module for more information.
[7]:
probe.shape
[7]:
(2, 1, 1, 2, 16, 16)
[8]:
probe *= 5 # reduce noise
[9]:
for m in range(probe.shape[-3]):
plt.figure()
tike.view.plot_phase(probe[0, 0, 0, m])
plt.show()
(-3.146963+3.8854375j) (4.9999995+0.0015707964j)
/home/dching/Documents/tike/src/tike/view.py:95: UserWarning: This phase plot will be incorrect because the phase of a zero-amplitude complex number is undefined. Adding a small constant to the amplitude may help.
"This phase plot will be incorrect because "
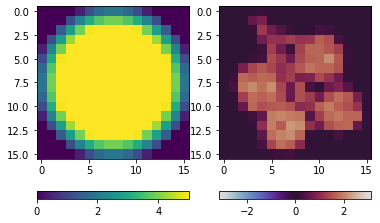
(-2.4711988+0.3783875j) (2.4927204+0.19064504j)
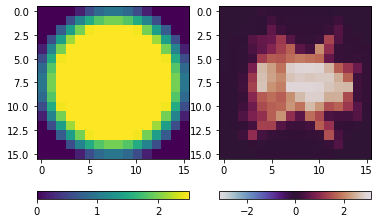
Information about a probe which varies with each scanning position is stored in the weights
and coherent_probe
arrays.
[10]:
# Add some probe variance
np.random.seed(0)
true_coherent_probe = probe
weights = 0.25 * tike.ptycho.probe.simulate_varying_weights(scan, true_coherent_probe)
true_probe = tike.ptycho.probe.get_varying_probe(probe, eigen_probe=true_coherent_probe, weights=weights)
[11]:
plt.figure()
plt.plot(weights[0, :, 0, 0])
plt.plot(weights[0, :, 0, 1])
[11]:
[<matplotlib.lines.Line2D at 0x7f23b95e8950>]
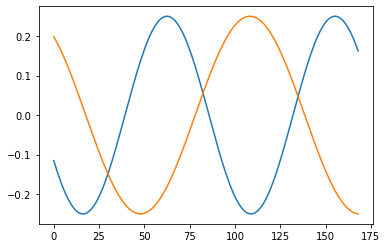
Define the trajectory¶
Each view may have a different trajectory, but the number of scan positions must be the same. The probe positions that overlap the edge of psi are not allowed.
[12]:
scan.shape
[12]:
(2, 169, 2)
[13]:
# Add some position noise
np.random.seed(0)
true_scan = scan + 1 * (2 * np.random.rand(*scan.shape) - 1)
[14]:
plt.figure()
plt.scatter(true_scan[0, :, 0], true_scan[0, :, 1])
plt.axis('equal')
plt.show()
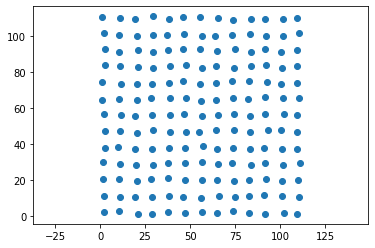
Simulate data acquisition¶
[15]:
# Then what we see at the detector is the wave propagation
# of the near field wavefront
data = tike.ptycho.simulate(detector_shape=probe.shape[-1] * 2,
probe=true_probe, scan=true_scan,
psi=original)
data.shape
[15]:
(2, 169, 32, 32)
[16]:
np.random.seed(0)
data = np.random.poisson(data)
[17]:
plt.figure()
plt.imshow(np.fft.fftshift(np.log(data[0, 11])))
plt.colorbar()
plt.show()
np.min(data), np.max(data)
/home/dching/miniconda3/envs/tike/lib/python3.7/site-packages/ipykernel_launcher.py:2: RuntimeWarning: divide by zero encountered in log
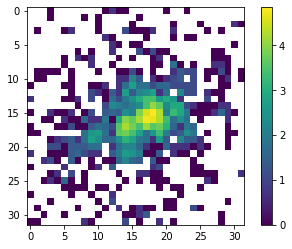
[17]:
(0, 665)
Reconstruct¶
Now we need to try and reconstruct psi.
[18]:
coherent_probe, weights = tike.ptycho.probe.init_varying_probe(scan, probe, 1)
[19]:
coherent_probe.shape, weights.shape
[19]:
((2, 1, 1, 2, 16, 16), (2, 169, 1, 2))
[20]:
# Provide initial guesses for parameters that are updated
np.random.seed(0)
result = {
'psi': np.ones(original.shape, dtype='complex64'),
'probe': probe * np.random.rand(*probe.shape),
'scan': scan,
'eigen_probe': coherent_probe,
'eigen_weights': weights,
}
[21]:
logging.basicConfig(level=logging.INFO)
result = tike.ptycho.reconstruct(
data=data,
**result,
algorithm='lstsq_grad',
num_iter=128,
recover_probe=True,
recover_psi=True,
)
INFO:tike.ptycho.ptycho:lstsq_grad for 169 - 32 by 32 frames for 128 iterations.
INFO:tike.ptycho.ptycho:object and probe rescaled by 1.398572
INFO:tike.ptycho.ptycho:lstsq_grad epoch 0
INFO:tike.ptycho.solvers.divided: farplane cost is +1.19073e+00
INFO:tike.ptycho.solvers.divided: farplane cost is +2.97681e-01
INFO:tike.ptycho.solvers.divided: nearplane cost is +2.50995e+02
INFO:tike.ptycho.solvers.divided: nearplane cost is +2.50995e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.20571e+02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.20571e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 1
INFO:tike.ptycho.solvers.divided: farplane cost is +8.44765e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +2.11191e-01
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.99078e+02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.99320e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.04930e+02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.05081e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 2
INFO:tike.ptycho.solvers.divided: farplane cost is +6.83100e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +1.70775e-01
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.70876e+02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.71403e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.59209e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.62758e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 3
INFO:tike.ptycho.solvers.divided: farplane cost is +5.95064e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +1.48766e-01
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.53957e+02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.54779e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.01969e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.07810e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 4
INFO:tike.ptycho.solvers.divided: farplane cost is +5.40806e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +1.35201e-01
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.42847e+02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.43959e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.62760e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.70968e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 5
INFO:tike.ptycho.solvers.divided: farplane cost is +5.03895e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +1.25974e-01
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.34963e+02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.36350e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.33744e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.44304e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 6
INFO:tike.ptycho.solvers.divided: farplane cost is +4.76868e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +1.19217e-01
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.29015e+02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.30661e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.10905e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.23766e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 7
INFO:tike.ptycho.solvers.divided: farplane cost is +4.55996e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +1.13999e-01
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.24317e+02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.26208e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.92119e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.07218e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 8
INFO:tike.ptycho.solvers.divided: farplane cost is +4.39239e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +1.09810e-01
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.20475e+02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.22598e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.76197e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.93470e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 9
INFO:tike.ptycho.solvers.divided: farplane cost is +4.25391e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +1.06348e-01
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.17251e+02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.19593e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.62398e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.81783e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 10
INFO:tike.ptycho.solvers.divided: farplane cost is +4.13688e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +1.03422e-01
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.14486e+02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.17038e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.50252e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.71690e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 11
INFO:tike.ptycho.solvers.divided: farplane cost is +4.03624e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +1.00906e-01
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.12075e+02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.14828e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.39437e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.62869e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 12
INFO:tike.ptycho.solvers.divided: farplane cost is +3.94844e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +9.87111e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.09943e+02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.12891e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.29719e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.55089e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 13
INFO:tike.ptycho.solvers.divided: farplane cost is +3.87097e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +9.67743e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.08037e+02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.11173e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.20910e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.48166e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 14
INFO:tike.ptycho.solvers.divided: farplane cost is +3.80194e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +9.50485e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.06319e+02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.09637e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.12864e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.41961e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 15
INFO:tike.ptycho.solvers.divided: farplane cost is +3.73992e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +9.34979e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.04758e+02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.08255e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.05470e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.36366e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 16
INFO:tike.ptycho.solvers.divided: farplane cost is +3.68378e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +9.20946e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.03331e+02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.07002e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.98637e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.31295e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 17
INFO:tike.ptycho.solvers.divided: farplane cost is +3.63267e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +9.08168e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.02020e+02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.05862e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.92292e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.26677e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 18
INFO:tike.ptycho.solvers.divided: farplane cost is +3.58587e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +8.96469e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.00811e+02
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.04820e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.86379e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.22459e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 19
INFO:tike.ptycho.solvers.divided: farplane cost is +3.54282e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +8.85704e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.96885e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.03863e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.80852e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.18599e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 20
INFO:tike.ptycho.solvers.divided: farplane cost is +3.50303e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +8.75757e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.86434e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.02980e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.75671e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.15063e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 21
INFO:tike.ptycho.solvers.divided: farplane cost is +3.46612e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +8.66529e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.76664e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.02164e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.70799e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.11818e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 22
INFO:tike.ptycho.solvers.divided: farplane cost is +3.43175e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +8.57937e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.67501e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.01407e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.66204e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.08838e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 23
INFO:tike.ptycho.solvers.divided: farplane cost is +3.39965e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +8.49911e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.58882e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.00704e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.61858e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.06101e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 24
INFO:tike.ptycho.solvers.divided: farplane cost is +3.36957e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +8.42392e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.50753e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +1.00049e+02
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.57736e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.03588e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 25
INFO:tike.ptycho.solvers.divided: farplane cost is +3.34130e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +8.35326e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.43068e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.94374e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.53816e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.01281e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 26
INFO:tike.ptycho.solvers.divided: farplane cost is +3.31468e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +8.28669e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.35786e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.88660e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.50079e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.99165e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 27
INFO:tike.ptycho.solvers.divided: farplane cost is +3.28953e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +8.22383e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.28872e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.83312e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.46508e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.97227e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 28
INFO:tike.ptycho.solvers.divided: farplane cost is +3.26572e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +8.16431e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.22293e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.78299e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.43088e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.95457e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 29
INFO:tike.ptycho.solvers.divided: farplane cost is +3.24314e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +8.10785e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.16021e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.73591e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.39805e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.93843e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 30
INFO:tike.ptycho.solvers.divided: farplane cost is +3.22167e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +8.05417e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.10029e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.69165e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.36648e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.92377e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 31
INFO:tike.ptycho.solvers.divided: farplane cost is +3.20121e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +8.00303e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.04296e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.64998e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.33606e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.91051e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 32
INFO:tike.ptycho.solvers.divided: farplane cost is +3.18170e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.95424e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.98799e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.61070e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.30672e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.89859e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 33
INFO:tike.ptycho.solvers.divided: farplane cost is +3.16304e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.90761e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.93519e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.57364e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.27839e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.88796e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 34
INFO:tike.ptycho.solvers.divided: farplane cost is +3.14519e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.86297e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.88441e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.53862e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.25102e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.87858e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 35
INFO:tike.ptycho.solvers.divided: farplane cost is +3.12807e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.82018e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.83549e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.50550e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.22455e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.87041e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 36
INFO:tike.ptycho.solvers.divided: farplane cost is +3.11165e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.77911e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.78829e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.47417e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.19893e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.86341e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 37
INFO:tike.ptycho.solvers.divided: farplane cost is +3.09586e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.73965e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.74271e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.44451e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.17413e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.85756e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 38
INFO:tike.ptycho.solvers.divided: farplane cost is +3.08068e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.70169e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.69864e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.41642e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.15010e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.85282e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 39
INFO:tike.ptycho.solvers.divided: farplane cost is +3.06606e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.66514e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.65598e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.38982e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.12679e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.84915e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 40
INFO:tike.ptycho.solvers.divided: farplane cost is +3.05196e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.62991e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.61467e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.36463e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.10418e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.84652e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 41
INFO:tike.ptycho.solvers.divided: farplane cost is +3.03837e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.59592e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.57462e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.34080e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.08222e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.84492e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 42
INFO:tike.ptycho.solvers.divided: farplane cost is +3.02524e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.56310e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.53577e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.31826e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.06088e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.84430e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 43
INFO:tike.ptycho.solvers.divided: farplane cost is +3.01255e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.53139e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.49805e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.29695e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.04011e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.84464e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 44
INFO:tike.ptycho.solvers.divided: farplane cost is +3.00028e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.50071e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.46142e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.27684e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.01990e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.84592e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 45
INFO:tike.ptycho.solvers.divided: farplane cost is +2.98840e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.47101e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.42582e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.25786e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.00019e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.84812e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 46
INFO:tike.ptycho.solvers.divided: farplane cost is +2.97689e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.44223e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.39119e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.24000e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.98098e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.85121e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 47
INFO:tike.ptycho.solvers.divided: farplane cost is +2.96573e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.41434e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.35749e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.22319e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.96223e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.85519e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 48
INFO:tike.ptycho.solvers.divided: farplane cost is +2.95491e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.38727e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.32468e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.20742e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.94392e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.86004e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 49
INFO:tike.ptycho.solvers.divided: farplane cost is +2.94439e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.36098e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.29272e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.19265e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.92603e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.86575e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 50
INFO:tike.ptycho.solvers.divided: farplane cost is +2.93418e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.33544e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.26156e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.17883e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.90854e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.87232e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 51
INFO:tike.ptycho.solvers.divided: farplane cost is +2.92424e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.31061e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.23118e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.16596e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.89143e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.87973e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 52
INFO:tike.ptycho.solvers.divided: farplane cost is +2.91458e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.28645e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.20154e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.15398e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.87468e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.88798e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 53
INFO:tike.ptycho.solvers.divided: farplane cost is +2.90517e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.26292e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.17259e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.14287e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.85828e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.89708e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 54
INFO:tike.ptycho.solvers.divided: farplane cost is +2.89600e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.24001e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.14432e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.13261e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.84221e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.90701e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 55
INFO:tike.ptycho.solvers.divided: farplane cost is +2.88707e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.21768e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.11669e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.12315e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.82647e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.91777e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 56
INFO:tike.ptycho.solvers.divided: farplane cost is +2.87836e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.19590e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.08966e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.11447e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.81105e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.92938e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 57
INFO:tike.ptycho.solvers.divided: farplane cost is +2.86986e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.17466e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.06322e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.10655e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.79593e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.94183e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 58
INFO:tike.ptycho.solvers.divided: farplane cost is +2.86157e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.15392e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.03734e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.09936e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.78110e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.95513e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 59
INFO:tike.ptycho.solvers.divided: farplane cost is +2.85347e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.13366e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.01199e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.09289e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.76657e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.96927e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 60
INFO:tike.ptycho.solvers.divided: farplane cost is +2.84555e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.11387e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.98715e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.08711e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.75231e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.98427e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 61
INFO:tike.ptycho.solvers.divided: farplane cost is +2.83781e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.09453e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.96280e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.08200e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.73832e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.00013e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 62
INFO:tike.ptycho.solvers.divided: farplane cost is +2.83024e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.07561e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.93893e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.07755e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.72459e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.01685e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 63
INFO:tike.ptycho.solvers.divided: farplane cost is +2.82284e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.05710e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.91551e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.07374e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.71110e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.03443e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 64
INFO:tike.ptycho.solvers.divided: farplane cost is +2.81559e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.03898e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.89253e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.07055e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.69786e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.05289e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 65
INFO:tike.ptycho.solvers.divided: farplane cost is +2.80849e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.02124e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.86998e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.06798e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.68485e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.07221e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 66
INFO:tike.ptycho.solvers.divided: farplane cost is +2.80154e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +7.00385e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.84782e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.06599e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.67206e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.09240e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 67
INFO:tike.ptycho.solvers.divided: farplane cost is +2.79473e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.98681e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.82607e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.06459e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.65948e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.11347e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 68
INFO:tike.ptycho.solvers.divided: farplane cost is +2.78804e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.97010e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.80468e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.06374e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.64710e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.13542e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 69
INFO:tike.ptycho.solvers.divided: farplane cost is +2.78149e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.95371e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.78366e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.06344e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.63491e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.15823e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 70
INFO:tike.ptycho.solvers.divided: farplane cost is +2.77505e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.93763e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.76299e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.06367e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.62291e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.18191e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 71
INFO:tike.ptycho.solvers.divided: farplane cost is +2.76873e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.92183e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.74265e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.06442e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.61108e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.20645e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 72
INFO:tike.ptycho.solvers.divided: farplane cost is +2.76253e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.90633e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.72263e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.06566e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.59941e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.23184e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 73
INFO:tike.ptycho.solvers.divided: farplane cost is +2.75643e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.89109e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.70293e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.06738e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.58791e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.25808e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 74
INFO:tike.ptycho.solvers.divided: farplane cost is +2.75044e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.87611e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.68353e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.06958e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.57656e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.28517e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 75
INFO:tike.ptycho.solvers.divided: farplane cost is +2.74455e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.86139e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.66442e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.07222e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.56536e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.31308e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 76
INFO:tike.ptycho.solvers.divided: farplane cost is +2.73876e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.84691e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.64559e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.07531e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.55429e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.34181e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 77
INFO:tike.ptycho.solvers.divided: farplane cost is +2.73306e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.83266e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.62703e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.07883e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.54336e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.37135e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 78
INFO:tike.ptycho.solvers.divided: farplane cost is +2.72746e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.81864e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.60874e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.08276e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.53256e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.40168e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 79
INFO:tike.ptycho.solvers.divided: farplane cost is +2.72194e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.80484e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.59069e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.08710e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.52189e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.43279e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 80
INFO:tike.ptycho.solvers.divided: farplane cost is +2.71650e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.79126e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.57289e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.09182e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.51134e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.46467e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 81
INFO:tike.ptycho.solvers.divided: farplane cost is +2.71115e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.77788e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.55533e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.09692e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.50090e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.49730e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 82
INFO:tike.ptycho.solvers.divided: farplane cost is +2.70588e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.76470e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.53799e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.10239e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.49058e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.53067e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 83
INFO:tike.ptycho.solvers.divided: farplane cost is +2.70068e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.75171e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.52087e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.10821e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.48037e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.56477e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 84
INFO:tike.ptycho.solvers.divided: farplane cost is +2.69556e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.73890e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.50396e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.11437e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.47027e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.59957e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 85
INFO:tike.ptycho.solvers.divided: farplane cost is +2.69051e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.72628e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.48726e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.12086e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.46028e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.63507e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 86
INFO:tike.ptycho.solvers.divided: farplane cost is +2.68553e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.71383e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.47076e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.12766e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.45039e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.67124e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 87
INFO:tike.ptycho.solvers.divided: farplane cost is +2.68062e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.70155e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.45444e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.13478e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.44059e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.70806e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 88
INFO:tike.ptycho.solvers.divided: farplane cost is +2.67577e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.68944e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.43831e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.14220e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.43090e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.74553e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 89
INFO:tike.ptycho.solvers.divided: farplane cost is +2.67099e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.67748e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.42236e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.14990e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.42130e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.78361e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 90
INFO:tike.ptycho.solvers.divided: farplane cost is +2.66627e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.66567e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.40659e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.15788e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.41178e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.82230e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 91
INFO:tike.ptycho.solvers.divided: farplane cost is +2.66161e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.65402e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.39098e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.16613e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.40236e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.86156e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 92
INFO:tike.ptycho.solvers.divided: farplane cost is +2.65700e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.64250e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.37554e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.17465e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.39301e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.90138e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 93
INFO:tike.ptycho.solvers.divided: farplane cost is +2.65245e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.63113e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.36026e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.18342e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.38375e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.94174e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 94
INFO:tike.ptycho.solvers.divided: farplane cost is +2.64796e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.61989e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.34514e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.19244e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.37457e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.98262e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 95
INFO:tike.ptycho.solvers.divided: farplane cost is +2.64351e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.60878e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.33017e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.20169e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.36546e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.02399e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 96
INFO:tike.ptycho.solvers.divided: farplane cost is +2.63912e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.59780e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.31534e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.21118e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.35643e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.06584e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 97
INFO:tike.ptycho.solvers.divided: farplane cost is +2.63478e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.58694e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.30066e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.22089e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.34748e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.10814e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 98
INFO:tike.ptycho.solvers.divided: farplane cost is +2.63048e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.57620e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.28613e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.23080e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.33859e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.15087e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 99
INFO:tike.ptycho.solvers.divided: farplane cost is +2.62623e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.56558e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.27173e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.24092e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.32978e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.19401e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 100
INFO:tike.ptycho.solvers.divided: farplane cost is +2.62203e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.55507e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.25747e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.25124e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.32103e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.23753e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 101
INFO:tike.ptycho.solvers.divided: farplane cost is +2.61787e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.54468e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.24335e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.26174e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.31235e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.28141e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 102
INFO:tike.ptycho.solvers.divided: farplane cost is +2.61376e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.53440e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.22937e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.27241e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.30374e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.32564e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 103
INFO:tike.ptycho.solvers.divided: farplane cost is +2.60969e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.52423e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.21552e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.28326e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.29519e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.37017e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 104
INFO:tike.ptycho.solvers.divided: farplane cost is +2.60566e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.51416e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.20180e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.29426e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.28670e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.41501e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 105
INFO:tike.ptycho.solvers.divided: farplane cost is +2.60168e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.50420e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.18820e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.30542e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.27828e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.46012e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 106
INFO:tike.ptycho.solvers.divided: farplane cost is +2.59774e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.49434e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.17474e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.31672e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.26992e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.50548e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 107
INFO:tike.ptycho.solvers.divided: farplane cost is +2.59383e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.48458e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.16140e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.32816e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.26162e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.55107e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 108
INFO:tike.ptycho.solvers.divided: farplane cost is +2.58997e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.47492e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.14818e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.33974e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.25339e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.59688e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 109
INFO:tike.ptycho.solvers.divided: farplane cost is +2.58614e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.46536e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.13508e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.35144e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.24522e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.64288e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 110
INFO:tike.ptycho.solvers.divided: farplane cost is +2.58236e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.45589e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.12210e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.36326e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.23712e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.68907e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 111
INFO:tike.ptycho.solvers.divided: farplane cost is +2.57861e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.44652e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.10924e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.37520e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.22909e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.73540e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 112
INFO:tike.ptycho.solvers.divided: farplane cost is +2.57490e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.43724e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.09649e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.38725e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.22113e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.78188e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 113
INFO:tike.ptycho.solvers.divided: farplane cost is +2.57122e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.42805e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.08386e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.39939e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.21324e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.82848e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 114
INFO:tike.ptycho.solvers.divided: farplane cost is +2.56758e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.41896e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.07134e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.41164e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.20542e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.87517e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 115
INFO:tike.ptycho.solvers.divided: farplane cost is +2.56398e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.40996e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.05893e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.42397e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.19766e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.92194e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 116
INFO:tike.ptycho.solvers.divided: farplane cost is +2.56042e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.40104e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.04663e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.43639e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.18998e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +8.96877e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 117
INFO:tike.ptycho.solvers.divided: farplane cost is +2.55689e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.39222e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.03443e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.44888e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.18236e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.01565e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 118
INFO:tike.ptycho.solvers.divided: farplane cost is +2.55340e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.38349e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.02234e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.46144e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.17482e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.06255e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 119
INFO:tike.ptycho.solvers.divided: farplane cost is +2.54994e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.37485e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +7.01035e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.47406e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.16736e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.10947e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 120
INFO:tike.ptycho.solvers.divided: farplane cost is +2.54652e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.36629e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.99846e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.48675e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.15997e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.15638e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 121
INFO:tike.ptycho.solvers.divided: farplane cost is +2.54313e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.35782e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.98668e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.49950e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.15265e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.20329e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 122
INFO:tike.ptycho.solvers.divided: farplane cost is +2.53978e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.34944e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.97500e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.51231e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.14540e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.25016e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 123
INFO:tike.ptycho.solvers.divided: farplane cost is +2.53646e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.34114e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.96342e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.52516e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.13823e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.29700e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 124
INFO:tike.ptycho.solvers.divided: farplane cost is +2.53317e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.33293e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.95195e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.53807e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.13113e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.34378e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 125
INFO:tike.ptycho.solvers.divided: farplane cost is +2.52992e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.32480e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.94059e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.55103e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.12410e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.39051e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 126
INFO:tike.ptycho.solvers.divided: farplane cost is +2.52670e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.31675e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.92933e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.56404e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.11713e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.43716e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.ptycho:lstsq_grad epoch 127
INFO:tike.ptycho.solvers.divided: farplane cost is +2.52351e-01
INFO:tike.ptycho.solvers.divided: farplane cost is +6.30879e-02
INFO:tike.ptycho.solvers.divided: nearplane cost is +6.91817e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.57709e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
INFO:tike.ptycho.solvers.divided: nearplane cost is +5.11023e+01
INFO:tike.ptycho.solvers.divided: nearplane cost is +9.48372e+01
INFO:tike.ptycho.solvers.divided:Updating eigen probes
[22]:
plt.figure()
plt.plot(result['cost'])
plt.show()
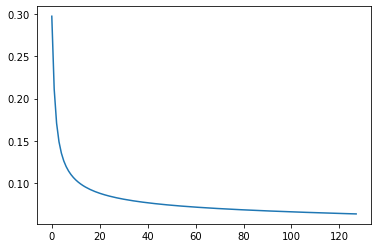
[23]:
for v in range(len(original)):
plt.figure(dpi=100)
tike.view.plot_phase(result['psi'][v], amin=0)
for m in range(probe.shape[-3]):
plt.figure(dpi=100)
tike.view.plot_phase(result['probe'][v, 0, 0, m], amin=0)
plt.show()
(-1.047305+0.17939374j) (3.7618608+0.655712j)
(-2.1428888+3.6436758j) (5.768509+0.8753591j)
(-3.153686+1.3175621j) (3.3205752+0.14948751j)
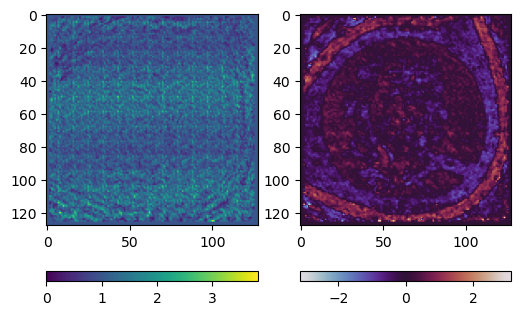
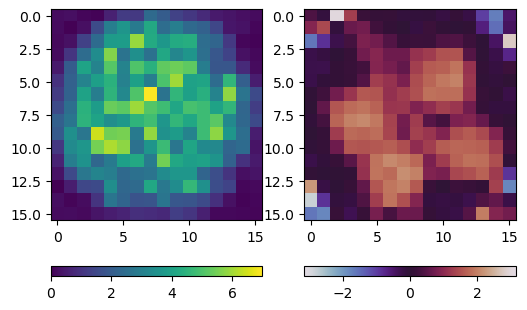
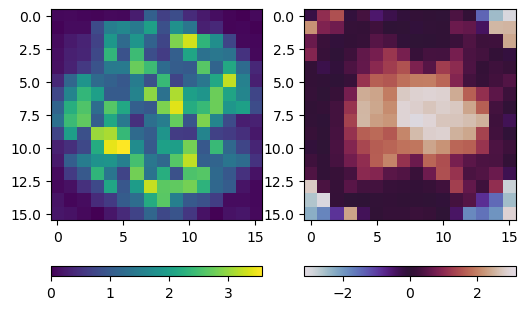
(-0.50873506+1.396821j) (3.1570942-0.3934597j)
(-3.0095506+5.134714j) (6.1496964+1.1832606j)
(-2.9979305+0.55080795j) (3.2052705+0.66596967j)
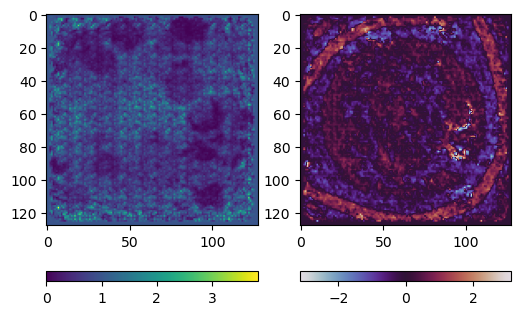
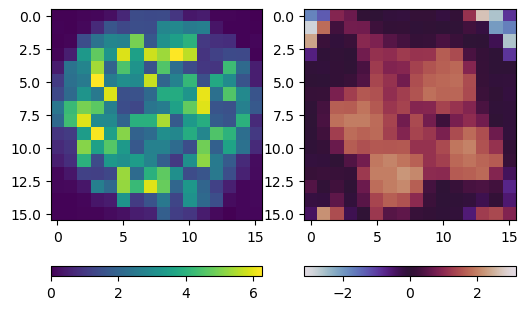
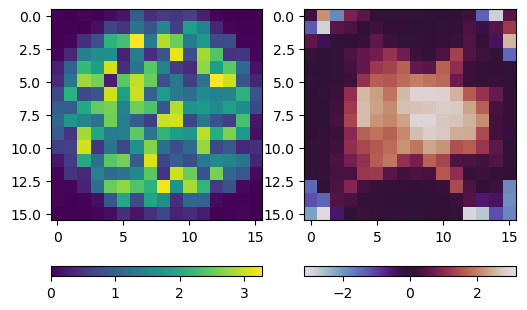
[ ]: