Ptychography Reconstruction#
This notebook demonstrates a ptychographic reconstruction using tike.
[ ]:
import logging
import matplotlib.pyplot as plt
import numpy as np
import cupy
import tike
import tike.ptycho
import tike.view
[2]:
for module in [tike, np, cupy]:
print("{} is version {}".format(module.__name__, module.__version__))
# Note the versions below were used to generate this notebook. Use a compatible version to get results shown here.
tike is version 0.25.1.dev4+gdf3f9c5
numpy is version 1.26.0
cupy is version 12.2.0
Load experimental data#
This data is a real dataset that has been pre-processed to be smaller so that it can be used as a test dataset. It contains the diffraction patterns, scan positions in pixel units, and an initial probe guess. There are functions in the tike.ptycho.io
module which may do this pre-processing automatically from select on-disk formats.
[3]:
import bz2
with bz2.open('../../../tests/data/siemens-star-small.npz.bz2') as f:
archive = np.load(f)
scan = archive['scan'][0]
data = archive['data'][0]
probe = archive['probe'][0]
The probe#
Note that the shape of the probe includes many dimensions. These leading dimensions are for providing multiple probes and to make internal multiplcations easier. This initial probe guess was generated from an analytical function which models the Freznel zone plate and other optics at the Velociprobe. Read the API documentation of the tike.ptycho.probe
module for more information.
[4]:
probe = tike.ptycho.probe.add_modes_random_phase(probe, 1)
[5]:
probe.shape, probe.dtype
[5]:
((1, 1, 1, 128, 128), dtype('complex64'))
[6]:
for m in range(probe.shape[-3]):
plt.figure()
tike.view.plot_phase(probe[0, 0, m])
plt.show()
(-0.22053738-0.014846489j) (0.21158107+0.0107072005j)
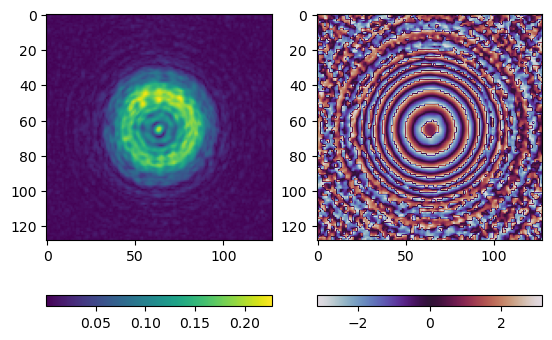
Define the trajectory#
The scanning positions must be scaled so that one scanning unit is the width of one pixel in the initial probe/object array; i.e. pixel units. All dimensions are consistently ordered; the scan[..., 0]
coordinates correspond to probe.shape[-2]
and data.shape[-2]
. A common pitfall of loading ptychographic data is that the dimensions are not consistent; be sure to try all combinations of swaping and flipping the sign(s) of the x and y axes.
If you provide an initial object (psi
), then you need to make it large enough to contain all of the scan positions plus the 2 pixel buffer along each edge plus the width of the probe in each dimension. If you do not provide an initial guess of the object, then tike does this shifting of the scanning positions automatically and will choose a conservatively large field of view.
[7]:
scan.shape, scan.dtype
[7]:
((516, 2), dtype('float32'))
[8]:
plt.figure()
plt.scatter(scan[:, 0], scan[:, 1])
plt.axis('equal')
plt.show()
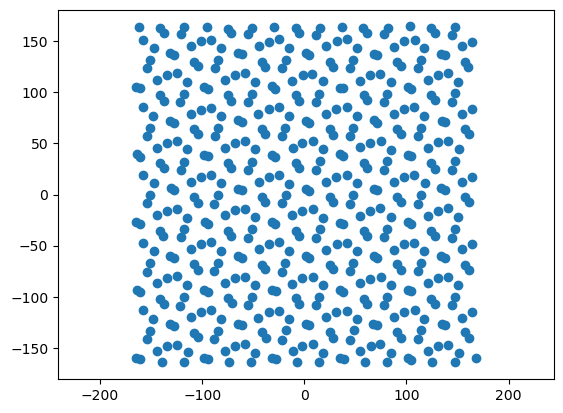
Diffraction patterns#
The diffraction patterns should be cropped or binned to a square shape that is larger than or equal to the shape of the probe array. Preferably, these arrays should be shaped to powers of 2 (for computational efficiency). The diffraction patterns should also be FFT shifted such that the zero-frequency (center of diffraction pattern) is at the origin. Bad pixels (NaNs, gaps between detector modules, known faulty pixels, …) should be set to zero or masked out of the reconstruction using ExitWaveOptions.
[9]:
plt.figure()
plt.imshow((data[11]))
plt.colorbar()
plt.show()
np.min(data), np.max(data), data.dtype
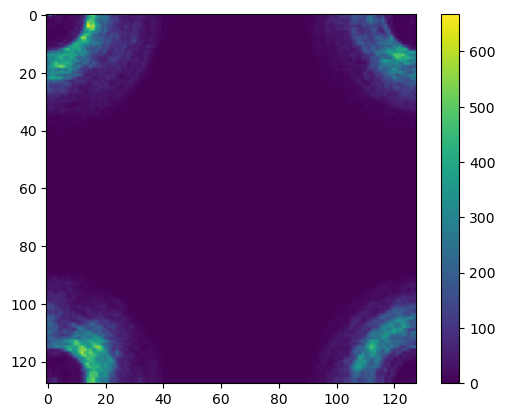
[9]:
(0.0, 963.0, dtype('float32'))
Reconstruct#
Now we try and reconstruct the object (psi
). To indicate which parameters we want to reconstruct, we use the Options classes. Providing no or None
Option indicates that a parameter will not be updated. Providing an empty Option indicates that the default options will be used.
[10]:
# initial guess for psi can be automatically generated, but it will recenter the scaning positions
psi, scan = tike.ptycho.object.get_padded_object(scan, probe)
[11]:
parameters = tike.ptycho.PtychoParameters(
# Provide initial guesses for parameters that are updated
probe=probe,
scan=scan,
psi=psi,
probe_options=tike.ptycho.ProbeOptions(), # uses default settings for probe recovery
object_options=tike.ptycho.ObjectOptions(
# The object will be updated.
use_adaptive_moment=True, # smoothness constraint will use our provided setting
# other object options will be default values
),
position_options=None, # indicates that positions will not be updated
algorithm_options=tike.ptycho.RpieOptions(
num_iter=64,
num_batch=7,
),
)
[12]:
logging.basicConfig(level=logging.INFO)
# returns an updated PtychoParameters object
result = tike.ptycho.reconstruct(
data=data,
parameters=parameters,
)
INFO:tike.ptycho.ptycho:rpie on 516 - 128 by 128 frames for at most 64 epochs.
INFO:tike.cluster:Clustering method is wobbly center.
INFO:tike.ptycho.ptycho:Probe rescaled by 188.669645
INFO:tike.ptycho.ptycho:rpie epoch 0
INFO:tike.ptycho.ptycho:The object update mean-norm is 9.964e-02
INFO:tike.ptycho.ptycho: gaussian cost is +3.270e+00
INFO:tike.ptycho.ptycho:rpie epoch 1
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.133e-01
INFO:tike.ptycho.ptycho: gaussian cost is +1.217e+00
INFO:tike.ptycho.ptycho:rpie epoch 2
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.191e-01
INFO:tike.ptycho.ptycho: gaussian cost is +9.556e-01
INFO:tike.ptycho.ptycho:rpie epoch 3
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.224e-01
INFO:tike.ptycho.ptycho: gaussian cost is +8.983e-01
INFO:tike.ptycho.ptycho:rpie epoch 4
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.248e-01
INFO:tike.ptycho.ptycho: gaussian cost is +8.684e-01
INFO:tike.ptycho.ptycho:rpie epoch 5
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.275e-01
INFO:tike.ptycho.ptycho: gaussian cost is +8.462e-01
INFO:tike.ptycho.ptycho:rpie epoch 6
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.303e-01
INFO:tike.ptycho.ptycho: gaussian cost is +8.279e-01
INFO:tike.ptycho.ptycho:rpie epoch 7
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.332e-01
INFO:tike.ptycho.ptycho: gaussian cost is +8.114e-01
INFO:tike.ptycho.ptycho:rpie epoch 8
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.361e-01
INFO:tike.ptycho.ptycho: gaussian cost is +7.961e-01
INFO:tike.ptycho.ptycho:rpie epoch 9
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.389e-01
INFO:tike.ptycho.ptycho: gaussian cost is +7.822e-01
INFO:tike.ptycho.ptycho:rpie epoch 10
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.328e-01
INFO:tike.ptycho.ptycho: gaussian cost is +7.689e-01
INFO:tike.ptycho.ptycho:rpie epoch 11
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.357e-01
INFO:tike.ptycho.ptycho: gaussian cost is +7.569e-01
INFO:tike.ptycho.ptycho:rpie epoch 12
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.386e-01
INFO:tike.ptycho.ptycho: gaussian cost is +7.454e-01
INFO:tike.ptycho.ptycho:rpie epoch 13
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.413e-01
INFO:tike.ptycho.ptycho: gaussian cost is +7.342e-01
INFO:tike.ptycho.ptycho:rpie epoch 14
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.438e-01
INFO:tike.ptycho.ptycho: gaussian cost is +7.228e-01
INFO:tike.ptycho.ptycho:rpie epoch 15
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.464e-01
INFO:tike.ptycho.ptycho: gaussian cost is +7.121e-01
INFO:tike.ptycho.ptycho:rpie epoch 16
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.488e-01
INFO:tike.ptycho.ptycho: gaussian cost is +7.019e-01
INFO:tike.ptycho.ptycho:rpie epoch 17
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.511e-01
INFO:tike.ptycho.ptycho: gaussian cost is +6.923e-01
INFO:tike.ptycho.ptycho:rpie epoch 18
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.534e-01
INFO:tike.ptycho.ptycho: gaussian cost is +6.825e-01
INFO:tike.ptycho.ptycho:rpie epoch 19
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.556e-01
INFO:tike.ptycho.ptycho: gaussian cost is +6.734e-01
INFO:tike.ptycho.ptycho:rpie epoch 20
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.568e-01
INFO:tike.ptycho.ptycho: gaussian cost is +6.646e-01
INFO:tike.ptycho.ptycho:rpie epoch 21
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.589e-01
INFO:tike.ptycho.ptycho: gaussian cost is +6.561e-01
INFO:tike.ptycho.ptycho:rpie epoch 22
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.609e-01
INFO:tike.ptycho.ptycho: gaussian cost is +6.477e-01
INFO:tike.ptycho.ptycho:rpie epoch 23
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.628e-01
INFO:tike.ptycho.ptycho: gaussian cost is +6.397e-01
INFO:tike.ptycho.ptycho:rpie epoch 24
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.647e-01
INFO:tike.ptycho.ptycho: gaussian cost is +6.319e-01
INFO:tike.ptycho.ptycho:rpie epoch 25
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.665e-01
INFO:tike.ptycho.ptycho: gaussian cost is +6.243e-01
INFO:tike.ptycho.ptycho:rpie epoch 26
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.682e-01
INFO:tike.ptycho.ptycho: gaussian cost is +6.171e-01
INFO:tike.ptycho.ptycho:rpie epoch 27
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.700e-01
INFO:tike.ptycho.ptycho: gaussian cost is +6.098e-01
INFO:tike.ptycho.ptycho:rpie epoch 28
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.716e-01
INFO:tike.ptycho.ptycho: gaussian cost is +6.031e-01
INFO:tike.ptycho.ptycho:rpie epoch 29
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.732e-01
INFO:tike.ptycho.ptycho: gaussian cost is +5.964e-01
INFO:tike.ptycho.ptycho:rpie epoch 30
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.740e-01
INFO:tike.ptycho.ptycho: gaussian cost is +5.899e-01
INFO:tike.ptycho.ptycho:rpie epoch 31
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.755e-01
INFO:tike.ptycho.ptycho: gaussian cost is +5.835e-01
INFO:tike.ptycho.ptycho:rpie epoch 32
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.770e-01
INFO:tike.ptycho.ptycho: gaussian cost is +5.775e-01
INFO:tike.ptycho.ptycho:rpie epoch 33
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.784e-01
INFO:tike.ptycho.ptycho: gaussian cost is +5.716e-01
INFO:tike.ptycho.ptycho:rpie epoch 34
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.798e-01
INFO:tike.ptycho.ptycho: gaussian cost is +5.659e-01
INFO:tike.ptycho.ptycho:rpie epoch 35
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.812e-01
INFO:tike.ptycho.ptycho: gaussian cost is +5.605e-01
INFO:tike.ptycho.ptycho:rpie epoch 36
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.825e-01
INFO:tike.ptycho.ptycho: gaussian cost is +5.552e-01
INFO:tike.ptycho.ptycho:rpie epoch 37
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.837e-01
INFO:tike.ptycho.ptycho: gaussian cost is +5.498e-01
INFO:tike.ptycho.ptycho:rpie epoch 38
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.850e-01
INFO:tike.ptycho.ptycho: gaussian cost is +5.448e-01
INFO:tike.ptycho.ptycho:rpie epoch 39
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.861e-01
INFO:tike.ptycho.ptycho: gaussian cost is +5.399e-01
INFO:tike.ptycho.ptycho:rpie epoch 40
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.867e-01
INFO:tike.ptycho.ptycho: gaussian cost is +5.352e-01
INFO:tike.ptycho.ptycho:rpie epoch 41
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.879e-01
INFO:tike.ptycho.ptycho: gaussian cost is +5.305e-01
INFO:tike.ptycho.ptycho:rpie epoch 42
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.890e-01
INFO:tike.ptycho.ptycho: gaussian cost is +5.260e-01
INFO:tike.ptycho.ptycho:rpie epoch 43
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.901e-01
INFO:tike.ptycho.ptycho: gaussian cost is +5.217e-01
INFO:tike.ptycho.ptycho:rpie epoch 44
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.911e-01
INFO:tike.ptycho.ptycho: gaussian cost is +5.175e-01
INFO:tike.ptycho.ptycho:rpie epoch 45
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.921e-01
INFO:tike.ptycho.ptycho: gaussian cost is +5.133e-01
INFO:tike.ptycho.ptycho:rpie epoch 46
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.931e-01
INFO:tike.ptycho.ptycho: gaussian cost is +5.093e-01
INFO:tike.ptycho.ptycho:rpie epoch 47
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.941e-01
INFO:tike.ptycho.ptycho: gaussian cost is +5.054e-01
INFO:tike.ptycho.ptycho:rpie epoch 48
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.950e-01
INFO:tike.ptycho.ptycho: gaussian cost is +5.015e-01
INFO:tike.ptycho.ptycho:rpie epoch 49
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.959e-01
INFO:tike.ptycho.ptycho: gaussian cost is +4.978e-01
INFO:tike.ptycho.ptycho:rpie epoch 50
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.963e-01
INFO:tike.ptycho.ptycho: gaussian cost is +4.941e-01
INFO:tike.ptycho.ptycho:rpie epoch 51
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.972e-01
INFO:tike.ptycho.ptycho: gaussian cost is +4.905e-01
INFO:tike.ptycho.ptycho:rpie epoch 52
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.980e-01
INFO:tike.ptycho.ptycho: gaussian cost is +4.872e-01
INFO:tike.ptycho.ptycho:rpie epoch 53
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.989e-01
INFO:tike.ptycho.ptycho: gaussian cost is +4.838e-01
INFO:tike.ptycho.ptycho:rpie epoch 54
INFO:tike.ptycho.ptycho:The object update mean-norm is 1.997e-01
INFO:tike.ptycho.ptycho: gaussian cost is +4.805e-01
INFO:tike.ptycho.ptycho:rpie epoch 55
INFO:tike.ptycho.ptycho:The object update mean-norm is 2.005e-01
INFO:tike.ptycho.ptycho: gaussian cost is +4.773e-01
INFO:tike.ptycho.ptycho:rpie epoch 56
INFO:tike.ptycho.ptycho:The object update mean-norm is 2.012e-01
INFO:tike.ptycho.ptycho: gaussian cost is +4.742e-01
INFO:tike.ptycho.ptycho:rpie epoch 57
INFO:tike.ptycho.ptycho:The object update mean-norm is 2.020e-01
INFO:tike.ptycho.ptycho: gaussian cost is +4.711e-01
INFO:tike.ptycho.ptycho:rpie epoch 58
INFO:tike.ptycho.ptycho:The object update mean-norm is 2.028e-01
INFO:tike.ptycho.ptycho: gaussian cost is +4.681e-01
INFO:tike.ptycho.ptycho:rpie epoch 59
INFO:tike.ptycho.ptycho:The object update mean-norm is 2.034e-01
INFO:tike.ptycho.ptycho: gaussian cost is +4.652e-01
INFO:tike.ptycho.ptycho:rpie epoch 60
INFO:tike.ptycho.ptycho:The object update mean-norm is 2.038e-01
INFO:tike.ptycho.ptycho: gaussian cost is +4.623e-01
INFO:tike.ptycho.ptycho:rpie epoch 61
INFO:tike.ptycho.ptycho:The object update mean-norm is 2.045e-01
INFO:tike.ptycho.ptycho: gaussian cost is +4.596e-01
INFO:tike.ptycho.ptycho:rpie epoch 62
INFO:tike.ptycho.ptycho:The object update mean-norm is 2.052e-01
INFO:tike.ptycho.ptycho: gaussian cost is +4.569e-01
INFO:tike.ptycho.ptycho:rpie epoch 63
INFO:tike.ptycho.ptycho:The object update mean-norm is 2.059e-01
INFO:tike.ptycho.ptycho: gaussian cost is +4.543e-01
[13]:
plt.figure()
plt.semilogy(result.algorithm_options.costs)
plt.show()
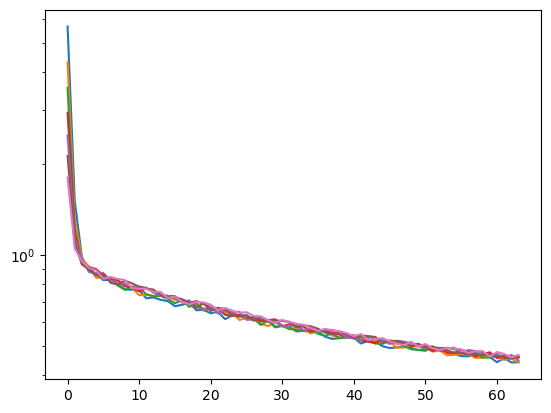
[14]:
plt.figure(dpi=200)
tike.view.plot_phase(result.psi, amin=0)
plt.show()
(-0.0877115+0.22840956j) (1.1543258+0.12585439j)
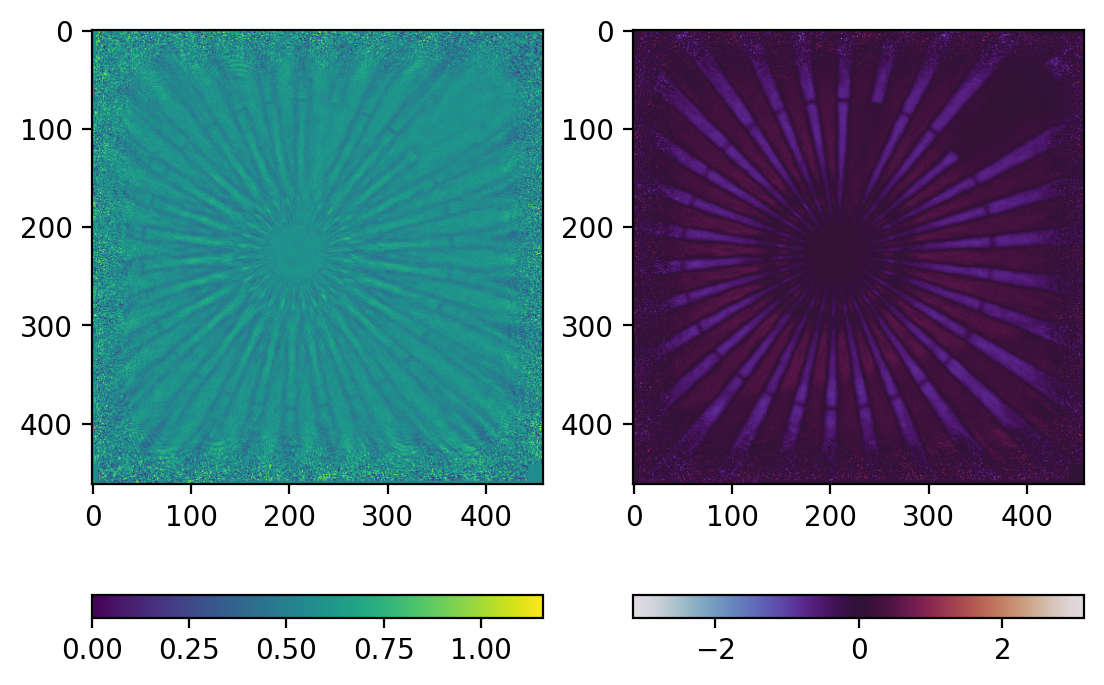
[ ]: